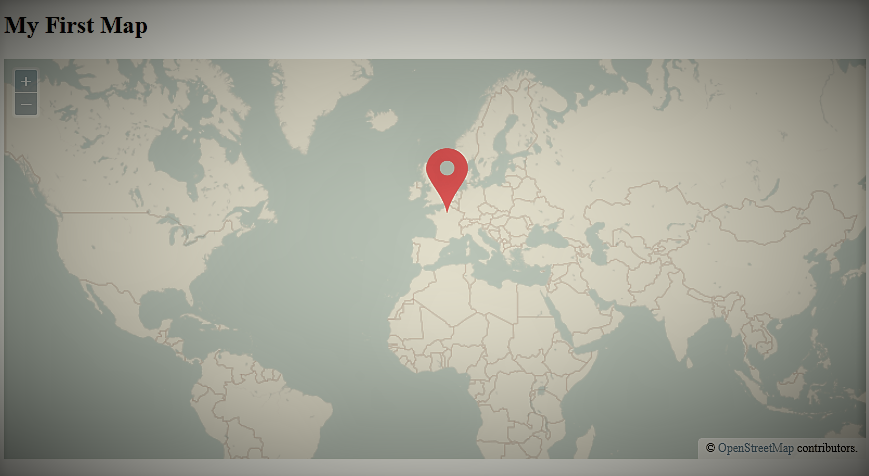
The simplest tutorial on creating a Map with Custom Markers with OpenLayers
Ok, let’s face it, Google has stopped giving things away for free.
There was a time when you could access most of their services including the Maps API. But nowadays, you need to cough up your credit card information upfront so that they can charge you when you use more than the free $200 quota. Sheesh Google.
Is there a free option?
Hmm… after searching a bit, I stumbled across OpenLayers. It is an Open Source, JavaScript based solution and is completely free (at least for now). Map data is available under the open database license.
There are no API keys that you need to setup and it’s rather quick to configure really.
Getting Started
Below, you’ll find the simplest way to get a map functioning. All you need is one puny file, nothing else. Save the following as a “.html” file and voila.
<!doctype html> <html lang="en"> <head> <meta charset="utf-8"> <link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/ol@v7.1.0/ol.css" type="text/css"> <script src="https://cdn.jsdelivr.net/npm/ol@v7.1.0/dist/ol.js"></script> <title>OpenLayers example</title> </head> <body> <div id="map" style="height: 400px;width: 100%;"></div> <script type="text/javascript"> var map = new ol.Map({ target: 'map', layers: [ new ol.layer.Tile({ source: new ol.source.OSM() }) ], view: new ol.View({ center: ol.proj.fromLonLat([2.2945, 48.8584]), zoom: 18 }) }); </script> </body> </html>
It will render like this in the browser. I’ve made some parts of the code bold. Play around with those to see what each parameter does to the map render.
I know, some explanation is in order.
This plain .html file works because it’s pulling 2 files from a CDN (Content Delivery Network). In this case, ol.js and ol.css files are being linked from a CDN called jsdelivr.net. Should you use a CDN or host them on your own website? That decision I leave it to you, since both have their advantages and disadvantages.
Adding markers
Just showing a plain map is just too little for most use-cases. What if you want to show the location of that fruit stand next to the Eiffel Tower? Just add the following snippet to the previous code in the <script> block. Make sure you have a marker.png file in the same folder as your .html file.
var markers = new ol.layer.Vector({ source: new ol.source.Vector(), style: new ol.style.Style({ image: new ol.style.Icon({ anchor: [0.5, 1], src: 'marker.png' }) }) }); map.addLayer(markers); var marker = new ol.Feature(new ol.geom.Point(ol.proj.fromLonLat([2.2931, 48.8584]))); markers.getSource().addFeature(marker);
There you go, you have a marker on the fruit stand. And just like that, you can add as many markers as you want to the map.
What next?
In one of the previous posts, I showed you how to do an IP Address Geo-location lookup to find location of an IP Address. You can now combine that with an OpenLayers map to show markers for various IP Addresses. You can, then for example, use this to show the location of visitors to your website. Hmm… that gives me an idea.
The following is a map of visitors to this website since the time of writing this article.